本文最后更新于:3 years ago
组合模式(Composite Pattern),又叫 部分整体模式 ,是用于把一组相似的对象当作一个单一的对象。组合模式依据树形结构来组合对象,用来表示部分以及整体层次。这种类型的设计模式属于结构型模式,它创建了对象组的树形结构。
这种模式创建了一个包含自己对象组的类。该类提供了修改相同对象组的方式。
如果想了解组合模式的具体的介绍,菜鸟教程介绍得比较详细↓
菜鸟教程-组合模式
结构图
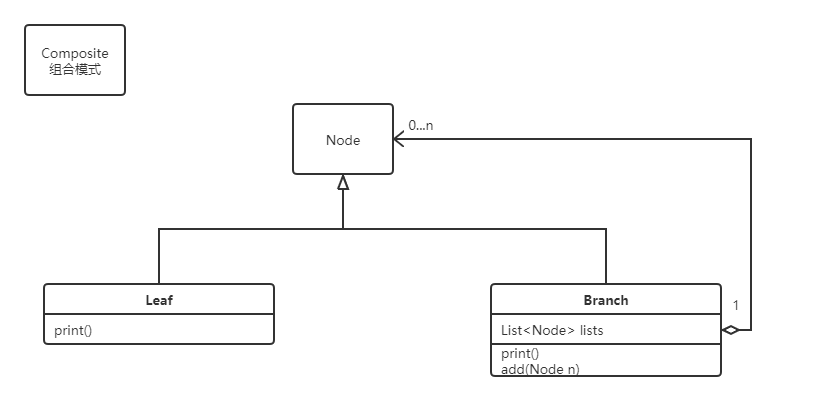
优缺点
优点:
1、高层模块调用简单。
2、节点自由增加。
缺点: 在使用组合模式时,其叶子和树枝的声明都是实现类,而不是接口,违反了依赖倒置原则。
使用场景
部分、整体场景,如树形菜单,文件、文件夹的管理。
实现代码
组合模式是很简单的模式,这里就模拟一下树的结构
先创建一个 Node
抽象类,里面有一个抽象的打印方法。
| abstract class Node { abstract public void p(); }
|
接着创建 LeafNode
类和 BranchNode
类来继承 Node
抽象类。
LeafNode
表示叶子节点,BranchNode
表示存在子节点的节点。
LeafNode
和 BranchNode
中都定义一个content,用来输出时表示这个节点是什么,
其中 BranchNode
中还定义了一个 List
类型的 nodes
用来存储 BranchNode
下面的节点。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| class LeafNode extends Node { String content;
public LeafNode(String content) { this.content = content; }
@Override public void p() { System.out.println(content); }
}
class BranchNode extends Node { List<Node> nodes = new ArrayList<>(); String content;
public BranchNode(String content) { this.content = content; }
@Override public void p() { System.out.println(content); }
public void add(Node node) { nodes.add(node); } }
|
最后就是写出主函数,我们创建一个根结点,然后根节点下面存在很多其他的子节点和叶子节点,
最后,用递归的方式完成输出:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| public class Main { public static void main(String[] args) { BranchNode root = new BranchNode("root"); BranchNode chapter1 = new BranchNode("chapter1"); BranchNode chapter2 = new BranchNode("chapter2"); Node c11 = new LeafNode("c11"); Node c12 = new LeafNode("c12"); BranchNode b21 = new BranchNode("section21"); Node c211 = new LeafNode("c211"); Node c212 = new LeafNode("c212");
root.add(chapter1); root.add(chapter2); chapter1.add(c11); chapter1.add(c12); chapter2.add(b21); b21.add(c211); b21.add(c212);
tree(root, 0); }
static void tree(Node b, int depth) { for (int i = 0; i < depth; i++) System.out.print("--"); b.p();
if (b instanceof BranchNode) { for (Node n : ((BranchNode) b).nodes) { tree(n, depth + 1); } } } }
|
输出结果:
| root --chapter1 ----c11 ----c12 --chapter2 ----section21 ------c211 ------c212
|